The Complete iOS 12 & 13 Masterclass Course: Build Real iOS Apps in Swift 4.2
What's Included
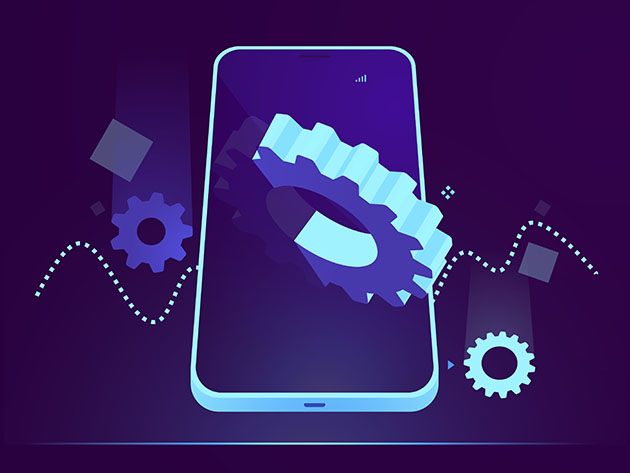
iOS 12 Masterclass Course
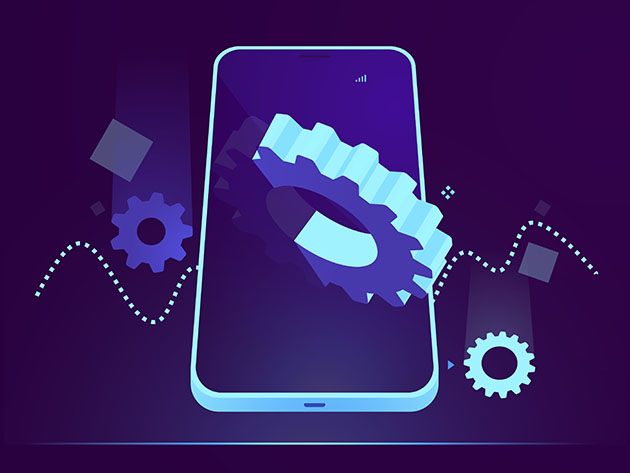
- Experience level required: All levels
- Access 246 lectures & 51 hours of content 24/7
- Length of time users can access this course: Lifetime
Course Curriculum
246 Lessons (51h)
- Your First Program
- IntroductionCourse Introduction2:20The Tools You Need1:08Installing Python 3 and an IDE6:43
- The Basics: Small ProgramYour First Python Code3:12Your first Python Program6:34SummaryFAQs
- The Basics: Data TypesVariables3:12Exercise: Assign Values and PrintSolution: Assign Values and PrintSimple Types: Integers, Strings, and Floats3:21List Types1:31Type attributes2:54How to find what code you need4:34Bonus: Steps of Learning Python1:28Dictionary Types3:43Tuple Types2:26How are datatypes used in the real world1:07Summary: Integers, Floats, Lists, Dictionaries, and TuplesExercise: Create Integers, Strings, and FloatsSolution: Create Integers, Strings, and FloatsExercise: Sum Up NumbersSolution: Sum Up NumbersExercise: Create ListSolution: Create ListExercise: Create Complex ListSolution: Create Complex ListExercise: Calculate MaximumSolution: Calculate MaximumExercise: Count ValuesSolution: Count ValuesExercise: Modify StringSolution: Modify String
- The Basics: Operations with Data TypesPython shell and terminal tips0:52More operations with lists5:56Accessing list items2:04Accessing list slices2:49Accessing items and slices with negative indexes2:02Accessing characters and slices in strings1:30Accessing items in dictionaries1:52Summary: Positive/Negative Indexes, Slicing
- The Basics: Functions and ConditionalsCreating Your Own Functions5:25Print or return3:59Intro to conditionals0:58If conditional example3:48Bonus Code: Using "and" and "or" in a ConditionalConditional explained line by line3:09More on conditionals2:21Elif conditionals1:15White space3:30Summary: Functions and Conditionals
- The Basics: Processing User InputUser input7:55String formatting2:44String formatting with multiple variables1:35Summary: Processing User Input
- The Basics: LoopsFor loops, how and why5:46For loop over a functionLooping through a dictionary1:12Bonus code: Dictionary loop and string formattingWhile loops: how and why2:59While loop example with user input3:12While loop with break and continue3:22Summary: Loops
- Putting the Pieces Together: Building a ProgramProblem statement3:48Approaching the problem1:46Building the maker function5:14Constructing the loop4:39Making the output user-friendly3:28
- List ComprehensionsSimple list comprehension3:27List comprehension with If conditional1:22List comprehension with if-else conditional1:37Summary: List Comprehensions
- More on FunctionsFunctions with multiple arguments1:45Keyword and non-keyword arguments, default and non-default parameters3:00Functions with an arbitrary number of non-keyword arguments3:32Functions with an arbitrary number of keyword arguments1:34Summary: More on Functions
- File ProcessingThe concept of processing files with Python1:12Reading text from a file3:07The cursor1:49Closing a file1:34Opening files using "with"1:45Different file paths1:25Writing text to a file3:59Appending text to an existing File3:30Summary: File Processing
- Imported ModulesBuiltin modules5:53Standard Python modules8:34Third-party modules5:49Third-party module example2:45Summary: Imported Modules
- Application 1: Build an Interactive DictionaryProgram demonstration4:10The data source4:54Loading JSON data3:52Returning the definition of a word3:25Counting for non-existing words2:51Implementing case sensitivity3:09Similarity ratio between two words4:39Best match out of a list of words6:07Recommending the best match9:42Confirmation from the user10:17Optimizing the final output7:51Exercise: Fixing a program bug (1)SolutionExercise: Fixing a program bug (2)Solution
- NumpyWhat is Numpy8:07Creating Numpy Arrays from Images and Vice-Versa12:30Indexing, Slicing and Iterating4:57Stacking and Splitting5:44
- Data Analysis with PandasWhat is Pandas6:37Installing PandasGetting Started with Pandas8:37Getting Started with Jupyter Notebooks9:18NoteLoading CSV Files4:20Exercise: Loading JSON FilesSolutionNote on Adding Excel FilesLoading Excel Files0:58Loading TXT Files2:30Set Header Row2:34Set Column Names0:56Set Index Column4:45Indexing and Slicing5:35Deleting Columns and Rows2:30Updating and Adding New Columns and Rows7:31Note on NominatimExample: Geocoding Addresses with Pandas and Geopy15:11
- Application 2: Create Webmaps with Python and FoliumDemonstration of the Web Mapping Application1:24Creating an Open Street Map with Python6:34Adding Markers to the Map5:10Adding Markers to the Map from CSV Data9:12Rule-based Coloring of Markers4:31More on Rule-based Styling4:27Calculating the Map Center from the Input Data7:56Adjusting the Code for the Latest Version of Folium8:12Adding a Choropleth Map from GeoJson20:59Adding a Layer Control Panel4:28
- Fixing Programming ErrorsSyntax errors8:22Runtime errors10:58Fixing difficult errors5:38The structure of a good programming question5:59Error handling7:59
- Application 3: Build a Website BlockerDemonstration of the Website Blocker Application3:48Application Architecture3:44Setting up the Script9:08Setting up the Infinite Loop11:00Implementing the First Part12:16Implementing the Second Part18:55Scheduling the Python Program on Windows12:39Scheduling the Python Program on Mac and Linux6:15
- Application 4: Build a Website with Python and FlaskDemonstration of the Website1:42Building Your First Website8:07Returning HTML Templates4:09Adding a Navigation Menu8:32Adding CSS Styling5:59Creating a Python Virtual Environment6:22Deploying the Website to a Live Server21:52Maintaining the Website7:26
- Graphical User Interfaces with TkinterIntroduction to Tkinter2:35Setting up a GUI with Widgets9:11Connecting GUI Widgets with Callback Functions9:33
- Interacting with Databases with PythonIntroduction to Working with Databases3:04Connecting and Inserting Data to SQLite via Python13:11Selecting, Inserting, Deleting, and Updating SQLite Records6:58Introduction to PostgreSQL Psycopg28:46Selecting, Inserting, Deleting, and Updating PostgreSQL Records12:53
- Application 5: Build a Desktop Database ApplicationDemonstration of the Database Application2:25User Interface Design5:54Building the Front-end Interface27:00Building the Back-end24:28Connecting the Front-end to the Back-end, Part 117:31Connecting the Front-end to the Back-end, Part 221:59Creating a Standalone Executable Version of the Program5:00
- Object Oriented ProgrammingObject Oriented Programming Explained4:59Turning this Application into OOP Style, Part 113:01Turning this Application into OOP Style, Part 214:06Creating a Bank Account Object21:06Inheritance12:08OOP Glossary8:12
- Python for Image and Video Processing with OpenCVIntroduction2:29Installing OpenCV for Python2:48Loading, Displaying, Resizing, and Writing Images with Python14:00Face Detection19:38Capturing Video19:45
- Application 6: Build a Webcam Motion DetectorDemonstration of the Motion Detector Application1:59Detecting Objects from the Webcam30:20Recording Motion Time20:38
- Interactive Data Visualization with PythonIntroduction to Bokeh2:02Installing BokehYour First Bokeh Plot13:52Plotting Triangles and Circle Glyphs (Practice)SolutionUsing Bokeh With Pandas4:51Plotting Education Data (Practice)SolutionNote on Loading Excel FilesPlot PropertiesPlot Weather Data (Practice)SolutionVisual AttributesTime-Series Plots6:36More Visualization Examples with Bokeh4:21Plotting Time Intervals of the Motion Detector14:05Hover Tool Implementation9:57
- Webscraping with Python Beautiful SoupSection Introduction1:57The Concept Behind Webscraping4:30Scraping a Webpage with Requests and BeautifulSoup16:22
- Application 7: Scrape Real Estate Property DataDemonstration of the Webscraping Application2:28Understanding the Problem and Loading the Webpage in Python7:15Extracting Divisions of All Properties11:34Extracting Addresses and Property Details14:39Extracting Elements with no Unique Identifiers12:07Saving the Extracted Data in CSV Files8:27Crawling Through Webpages17:15
- Application 8: Build a Web-based Financial GraphDemonstration of the Financial Analysis Application1:59Downloading Various Datasets with Python11:31Understanding Stock Market Data3:25Understanding Stock Market Data Candlestick Charts5:39Building Chart Candlesticks with Bokeh Quadrants10:13Building Chart Candlesticks with Bokeh Rectangles22:28Building Candlestick Segments5:02Stylizing the Chart4:21The Concept Behind Embedding a Bokeh Chart in a Webpage11:04Embedding the Bokeh Chart in a Webpage15:33Deploying the Chart Website to a Live Server8:22
- Application 9: Build a Data Collector Web AppDemonstration of the Web Application2:59Steps for Building a PostgreSQL Database-enabled Web Application6:08Building the Front-end: HTML Part14:52Building the Front-end: CSS Part10:11Building the Back-end: Getting User Input17:31Building the Back End: Creating the PostGreSQL Database Model18:17Building the Back End: Storing User Data to the Database19:14Building the Back End: Emailing Database Values Back to the User11:14Building the Back End: Sending Statistics to Users16:00Deploying the Web Application to a Live Server29:31Bonus Lecture: User Downloads and Uploads20:51
- Application 10: Student Project on Building a Geocoder Web ServiceDemonstration of the Geocoding Web Service Application and Project Requirements7:31Solution, Part 116:21Solution, Part 25:51End of the Course0:47
iOS 12 Masterclass Course
Jonathan Burgoyne | iOS & Android Developer | Teacher
Description
Absorbing theories and concepts on mobile development adds vital knowledge, but learning through actual practice is something else. This course teaches you how to build beautiful iOS 12 apps using the latest in Swift 4.2. This masterclass is packed with 51 expert-led lectures on building real iOS apps to build your portfolio, worthy of submission to the App Store. It covers Apple's updated ARKit 2 for building Augmented Reality apps as well as Core ML 2 & Create ML for creating apps that think with Machine Learning. You'll generate your own machine learning models and build 3D augmented reality apps. By the end of this course, you'll have 15+ apps for your portfolio.
- Access 246 lectures & 51 hours of content 24/7
- Understand basic to intermediate iOS development
- Learn how to build apps w/ Firebase
- Develop Augmented Reality apps using the newest ARKit 2 framework
Specs
Important Details
- Length of time users can access this course: lifetime
- Access options: web & mobile streaming
- Certification of completion included
- Redemption deadline: redeem your code within 30 days of purchase
- Updates included
- Experience level required: all levels
- Have questions on how digital purchases work? Learn more here
Requirements
- Internet access required
- macOS High Sierra or Mojave
- Xcode 10 installed
Terms
- Unredeemed licenses can be returned for store credit within 30 days of purchase. Once your license is redeemed, all sales are final.
Kiran Sasidharan
Good.New learning experience.New input.Might benefit with new to this area.Learning helps to advance in general.